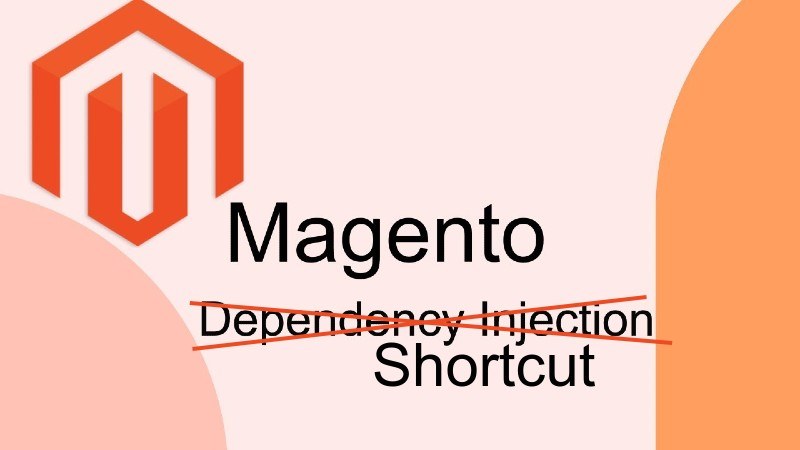
How to call any block in any phtml template in Magento 2
In Magento 2, it's possible to call any block into any phtml with two lines of code. Ideally, dependency injection should be used but I'll demonstrate both methods. I will use FirstModule and SecondModule to distinguish between the two modules.
The correct way
In the Block construct for your FirstModule phtml:
namespace Vendor\FirstModule\Block; class Custom extends Template { private $custom; public function __construct( ... \Vendor\SecondModule\Block\Custom $custom ... ) { ... $this->custom = $custom; ... } public function getCustomMethod() { // This references the "SecondModule" Custom class $custom = $this->custom; // Returns the getCustomMethod() from the "SecondModule" return $custom->getCustomMethod(); }
In the FirstModule phtml where the SecondModule "getCustomMethod" output will be displayed:
<?= $block->getCustomMethod(); ?>
The not-as-correct way that also works (no injection)
In the FirstModule phtml where the custom SecondModule output will be displayed:
<?php $customBlock = $block->getLayout()->createBlock('Vendor\SecondModule\Block\Custom'); echo $customBlock->getCustomMethod(); ?>